Make a TIN inspector with Python,Using a point monitor
from pyrx_imp import Rx
from pyrx_imp import Ge
from pyrx_imp import Gi
from pyrx_imp import Db
from pyrx_imp import Ap
from pyrx_imp import Ed
from pyrx_imp import Gs
from pyrx_imp import Cv
import traceback
print("added command tininspecton")
print("added command tininspectoff")
# exit clean
def OnPyUnloadApp():
PyRxCmd_tininspectoff()
class MyPointMonitor(Ed.InputPointMonitor):
def __init__(self):
Ed.InputPointMonitor.__init__(self)
self.line = Db.Line()
self.face = Db.Face()
self.face.setColorIndex(1)
def draw(self, input, plcv, viewDir):
#filtype didn't work just draw a face, draw on top
self.face.setVertexAt(0,plcv[0]+ viewDir)
self.face.setVertexAt(1,plcv[1]+ viewDir)
self.face.setVertexAt(2,plcv[2]+ viewDir)
self.face.setVertexAt(3,plcv[0]+ viewDir)
input.drawContext().geometry().draw(self.face)
def monitorInputPoint(self, input, output):
try:
ents = input.pickedEntities()
if len(ents) == 0:
return
if not ents[0].isDerivedFrom(Cv.CvDbTinSurface.desc()):
return
viewDir = Ed.Core.getVar("VIEWDIR").asVector()
tin = Cv.CvDbTinSurface(ents[0])
self.line.setStartPoint(input.rawPoint())
self.line.setEndPoint(input.rawPoint() + viewDir * tin.maxElevation())
pnts = tin.intersectWith(self.line, Db.Intersect.kExtendArg)
if len(pnts) == 0:
return
tris = tin.findTinTrianglesAt(pnts[0])
if len(tris) == 0:
return
tri: Cv.CvTinTriangle = tris[0]
if not tri.isValid():
return
plcv = [
tri.pointAt(0).location(),
tri.pointAt(1).location(),
tri.pointAt(2).location()
]
# tooltip, use elev at point?
zs = [p.z for p in plcv]
tip = "Min Elev = {:.2f}\nMax Elev = {:.2f}".format(min(zs),max(zs))
output.setAdditionalTooltipString(tip)
self.draw(input,plcv,viewDir)
except Exception as err:
print(err)
pm = MyPointMonitor() #global
def PyRxCmd_tininspecton():
try:
Ap.curDoc().inputPointManager().addPointMonitor(pm)
except Exception as err:
traceback.print_exception(err)
def PyRxCmd_tininspectoff():
try:
Ap.curDoc().inputPointManager().removePointMonitor(pm)
except Exception as err:
traceback.print_exception(err)
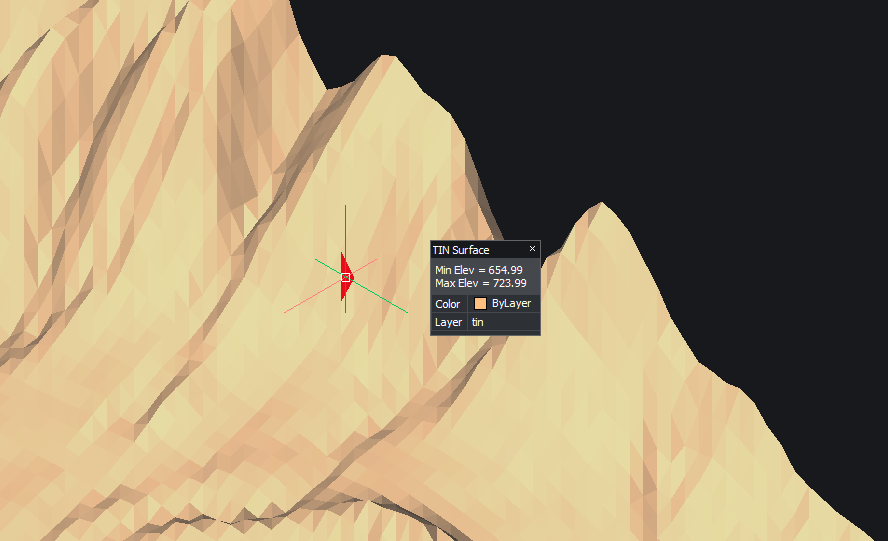
0
Comments
-
Access to the triangles is really fast, well, the set only has 50,000 points
0 -
Cool, only question is how do you run python I know about Pyacad but that is it.0
-
Python wrappers for BRX are in progress here, https://github.com/CEXT-Dan/PyRx
The ActiveX APIs are also mostly wrapped. wxPython/wxWidgets is the GUI framework
It’s all in process, so the performance is close to .NET
It was rather difficult to port to BRX, so only V24 Pro and later versions
0